C# is a strongly typed and object-oriented programming language developed and launched by Microsoft in 2001. It is simple and modern that gives developers flexibility and features to create software that are future-proof.
Lets start with our hello world program in c#. Open visual studio and go to File > New > Project.
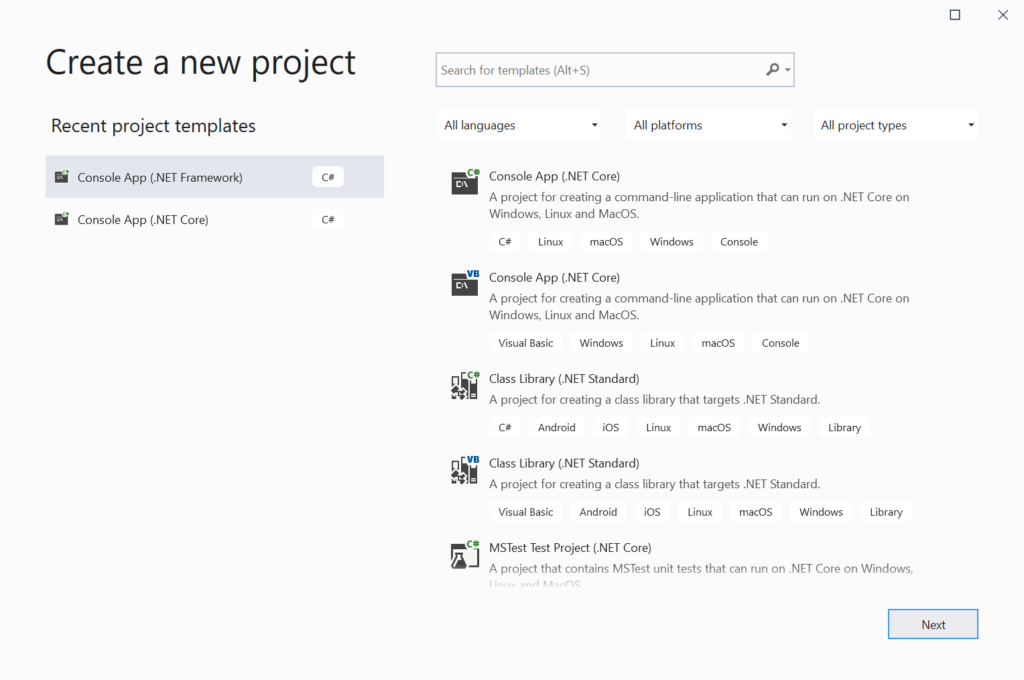
In the search bar type Console App

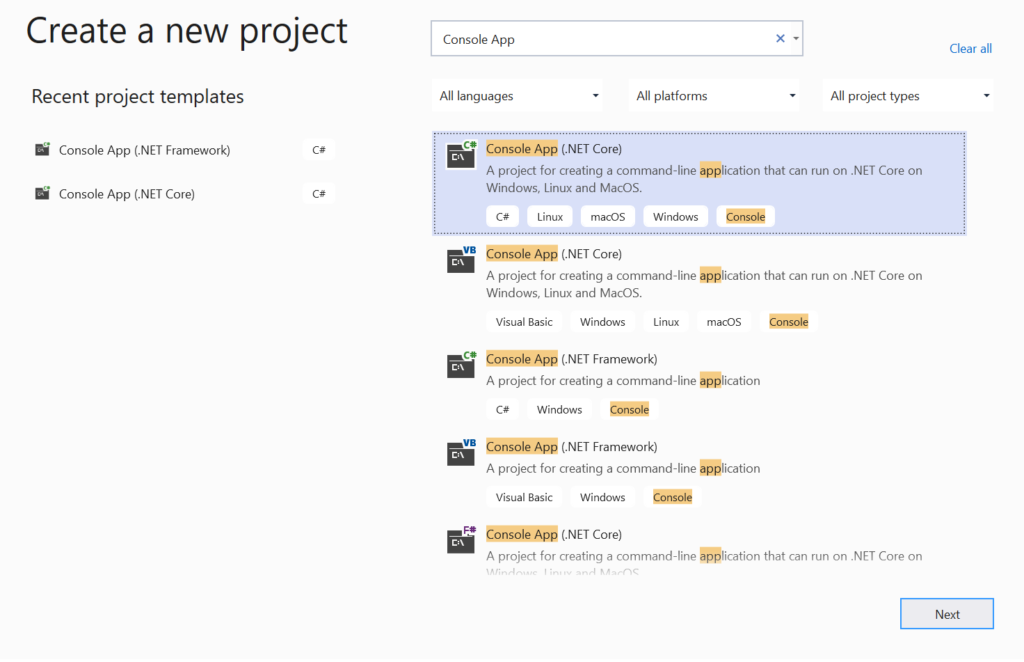
If you can not find it, don't worry!
Scroll down until you see Not Finding what you’re looking for?
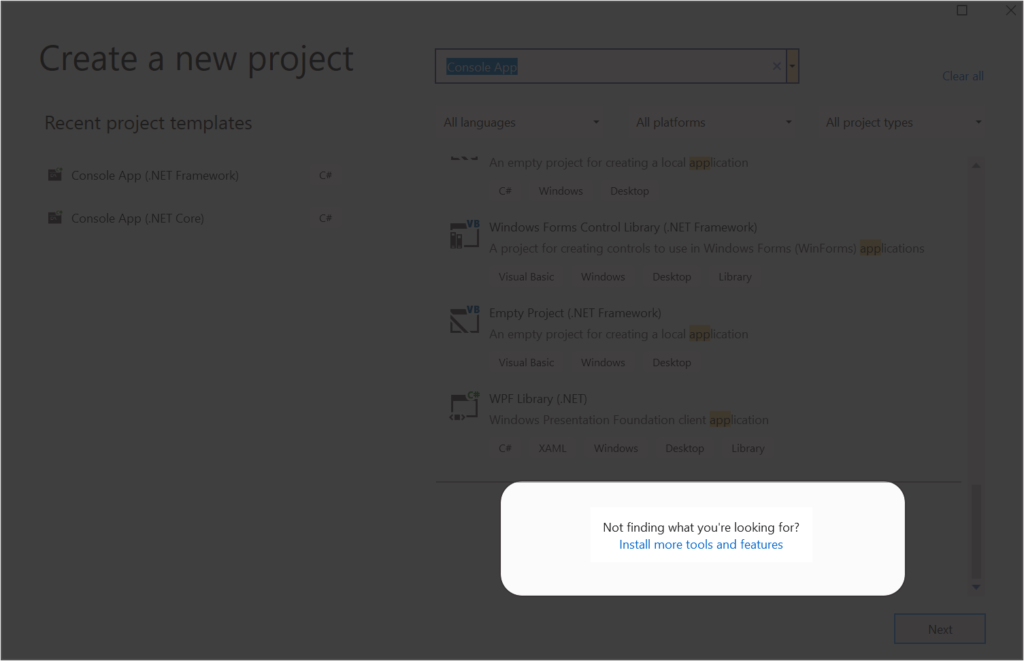
In Visual Studio Installer choose the .NET Core cross-platform development workload and Install it.
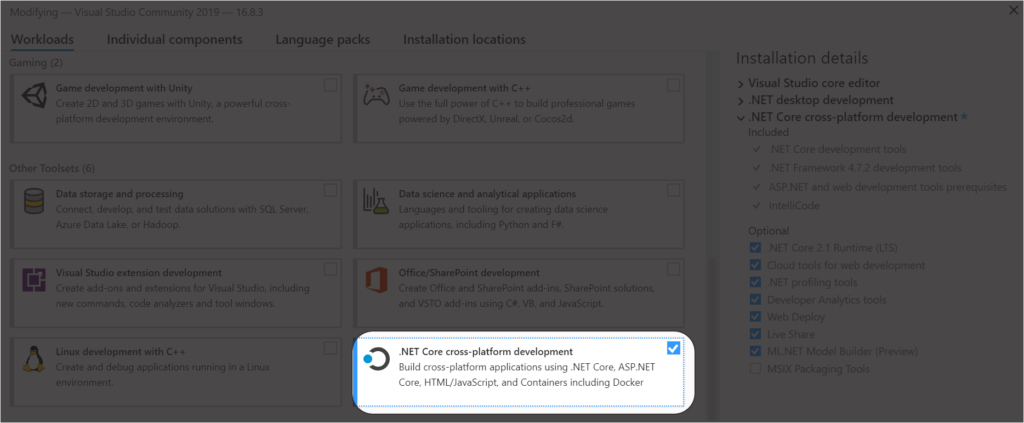
Create a new Console App project name it whatever you like. I named it ConsoleApp1, yeah I am not good with naming.
On the right side you will see Solution Explorer. If you don’t see it go to View > Solution Explorer.
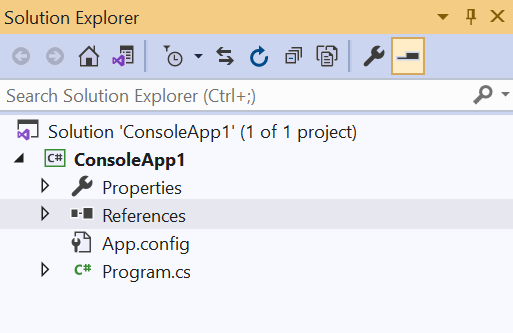
First folder you is Properties. It consist of one file called AssemblyInfo.cs. It has all the build options for your project such as Title, Description, Copyright and versioning and much more. These all are part of assembly identification or Assembly Manifest. In most case, you don’t have to worry about it. But if you are creating a project that will be distributed to other people, then you might want to come to this file and give it proper name, description, version and other attributes.
In reference folder you add/manage components or assemblies that your project will be using. These components can be developed by Microsoft of other companies. By default you get some assemblies are added by visual studio.
App.config is an XML file where we store the configuration of our application. We might store connection strings to Database or Application settings, all of these will come in this file.
The last folder that you will see is Programe in it we have a file called Programe.cs in which we will start writing the code.
Let’s go through this file. On the top you will see some statements with word using
in the beginning of it.
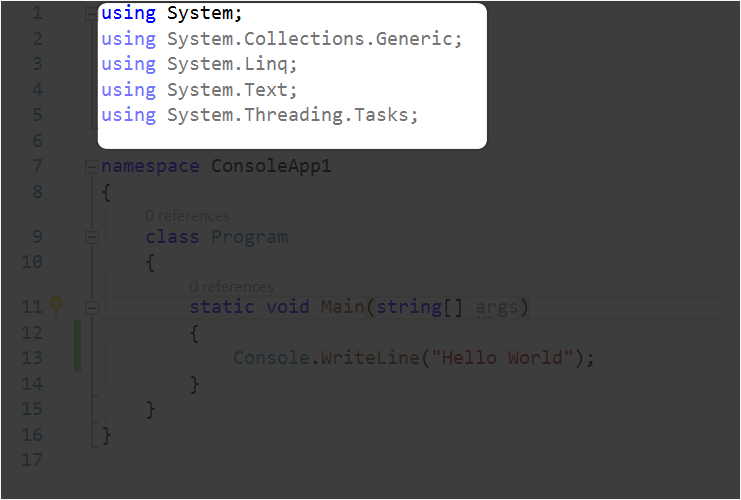
When we create our application, Visual Studio will create a namespace with the name of the project. Now in that namespace whatever out side components or assemblies we want to use we will have to import them by using the key world `using`. You might have noticed that some lines are greyed out, that is because we are not using them in program. You can actually delete them, as we will not be using it in our hello world program. In visual studio you can delete a line by having the cursor in that line and enter CTRL + x. We can also delete all the unnecessary Usings by having the cursor in one of those Usings and enter ALT + ENTER and click on Remove Unnecessary Usings. (see the image below)
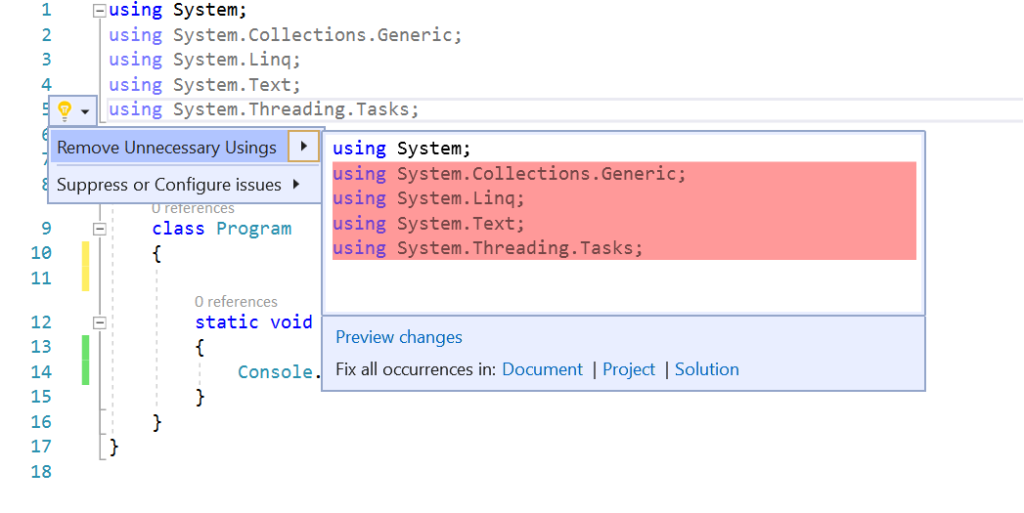
When our project is created, the visual studios creates a namespace that contains Class Program. In Program class, by default we have a method or function Main. It is the entry point to the application. When we run our application CLR executes the method in this Main method. We will learn about Main methods types and it’s parameters in the coming tutorials.
We will write a simple code that will print “Hello World” when executed.
using System;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World");
}
}
}
You can run your code now by entering CTRL + F5.
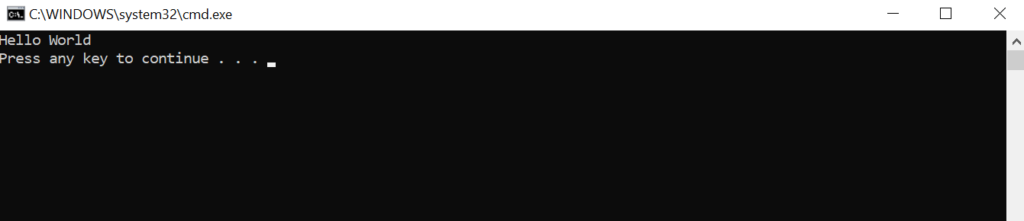